How To Solve Coding Interview Questions (An Ultimate Guide)
Solving coding interview questions is the act of working through the types of problems that are asked during a software engineer technical interview. The problems that are asked during a technical interview (also known as a coding interview) are typically questions that require you to implement either a function or class structure in a popular programming language like Python, Java, C++, or JavaScript.
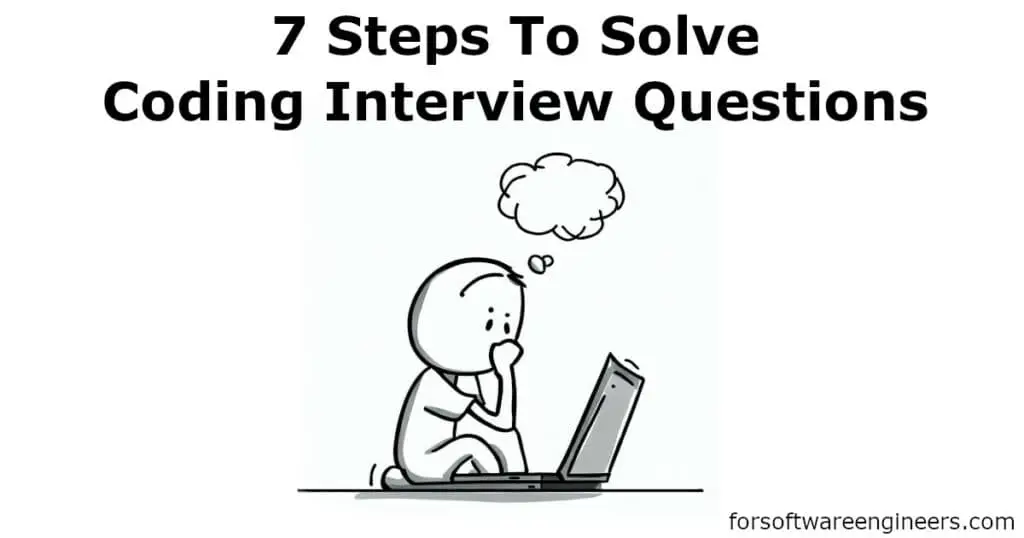
It’s important that you understand how to solve a coding interview problem optimally, and it is also important to understand what your interviewer requires you to do. Both aspects of solving a technical interview problem are important if your goal is to pass a coding interview.
There are methods to approaching solving coding interviews like the UMPIRE method (understand, match, plan, implement, review, and evaluate), but we suggest a methodology that enables you to emit more positive evaluation signals during an interview process.
To solve coding interview questions for the purpose of passing a coding interview, you will need to follow the instructions listed below (in the order they’re listed).
- Understand the provided question
- Determine the solution space
- Analyze the solution space (in terms of big O)
- Confirm a solution to code with your interview
- Code your first solution
- Test your code
- Optimize your solution
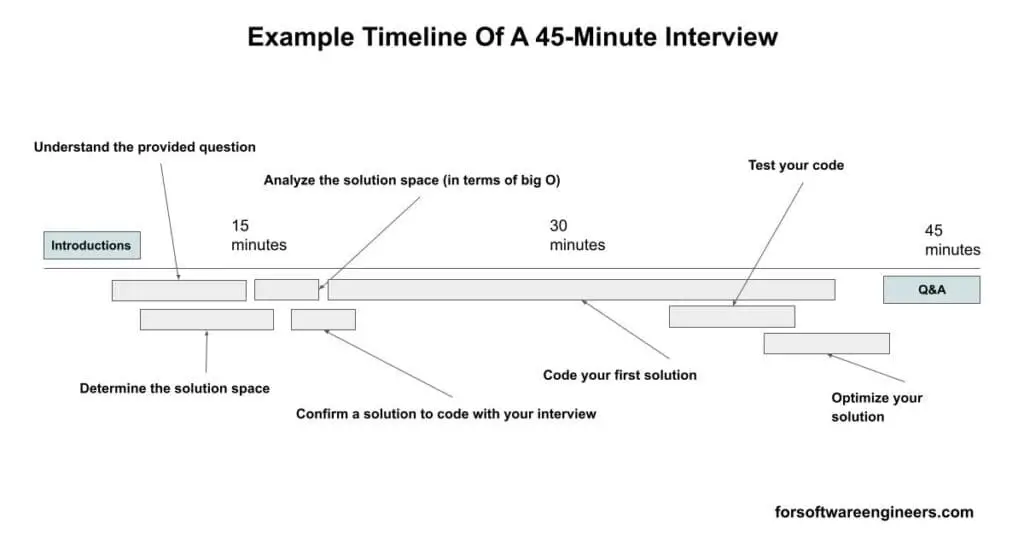
In the sections below, we will elaborate on what each of these steps requires you to do in order to solve technical interview problems with the intention of doing well in an interview. At the end of the article, we will discuss additional ways you can improve your chances of passing a coding interview.
1. Understand The Provided Question
The first step to solving a coding interview problem is to understand the provided question you’re asked. If you don’t fully understand the question before you start thinking about and coding solutions then you’re automatically going to fail to solve the problem.
Understanding the provided question means making sure that you understand what the provided interview question expects you to do based on its inputs, outputs, and constraints. Detailed explanations of inputs, outputs, and constraints for solving coding interview problems are listed below.
- Inputs: aspects of a coding problem to solve such as the method signature to a function you’re implementing and any helper functions (or classes) that your interviewer also provides you with to help you solve the problem.
- Outputs: aspects of a coding interview problem in terms of what the output of your code should be.
- Constraints: bounds for your inputs which depend on the type of your input. For instance, a constraint might be that your input will be a number between 1 and 100, so you don’t have to account for numbers outside that bound in your code.
You should ask clarifying questions that relate to the inputs, outputs, and constraints, to demonstrate that you understand the provided question to your interviewer. Clarifying questions are questions that you ask your interviewer for more detailed explanations of a specific aspect of an interviewer’s question. Additionally, you should run through examples of inputs and outputs, to further clarify your understanding of a problem.
What Are Good Clarifying Questions To Ask?
Good clarifying questions to ask include questions that are related to the inputs and outputs in terms of edge cases. Many interview candidates are able to successfully understand the inputs and outputs of basic cases, but often they forget to account for edge cases in inputs and what to return for edge cases within the code.
Examples of clarifying questions are listed below.
- Do I have to worry about null inputs or null outputs?
- How should I represent empty return types (i.e., null, empty list, empty object)?
- Do I have to make sure my solution covers <edge case>?
2. Determine The Solution Space
The second step to solving a coding interview during an interview is to ensure that you’re able to determine the solution space.
Determining the solution space when solving a technical interview problem means determining what the possible correct solutions are for a problem that you can implement. Typically, there’s an easy-to-identify brute force solution (a solution that is inefficient in terms of time and space, but easy to implement). However, interviewers typically don’t want you to implement a brute-force solution.
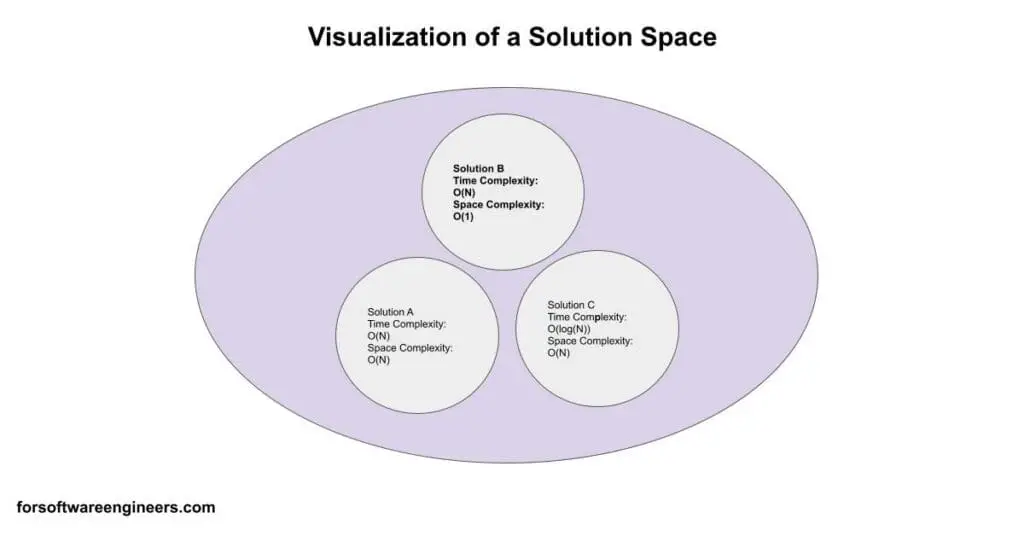
Your solution space should be determined before you start writing code. If you start writing code before finishing your solution space, your interviewer will likely consider that a negative signal toward your evaluation as a candidate because it expresses that you don’t think through solutions before implementing them.
How Do You Ideate Potential Solutions?
To ideate solutions when solving a coding interview problem, you should think about the best data structures and algorithms that can be used within the programming problem you’re asked. To do this, you should go through examples of solving the problem with your interviewer to identify patterns in the solution that map to specific data structures and algorithms.
Additional tips for ideating potential solutions when solving interview problems are listed below.
- Visualize the problem: Illustrating what a solution to a problem looks like based on a problem statement is often easier than trying to think about the implementation in code. You can try to solve the problem by hand on a whiteboard (if interviewing in person) or in text within an IDE (if interviewing online)
- Break the problem down into smaller parts: Often, a problem can be split into smaller implementations that build up into a larger problem. Breaking a problem into sub-problems is a great way to ideate solutions that are more efficient.
- Ask for hints from the interviewer: While you should try to avoid directly asking your interviewer for hints, you can ask the interviewer for guidance if you’re stuck on finding more optimal solutions. If you are asking for hints, you should explain to your interviewer what you’ve already thought of and what you’re currently thinking of so they can understand your thought process.
3. Analyze The Solution Space (Big O)
After you have your solution space, the next step is to analyze your solution space in terms of Big O time and space complexity. Time and space complexity is how software engineers compare the efficiency of programs at scale by understanding how efficient a program is in its worst case.
The table below shows the worst-case time and space complexities of a solution space to the LeetCode problem called Two-Sum.
Solution Description | Worst-case Time Complexity | Worst-case Space Complexity |
Brute Force | O(n2) | O(1) |
Two-pass Hash Table | O(n) | O(n) |
One-pass Hash Table | O(n) | O(n) |
Often, candidates either forget to mention the time and space complexities of the solutions or explain the time and space complexity after coding a solution. But remember, time and space complexity is an important aspect of solving the coding interview with the intention of passing the coding interview, so you should not forget to discuss this.
Once you’re able to describe the solutions’ time and space complexities in Big O notation, make sure that you explain to your interviewer “why” each of the solutions should be the time and space complexities you’ve mentioned.
This can be considered difficult to do because you haven’t coded a solution yet, but you should at least communicate to your interviewer an education prediction of each solution’s time and space complexities which requires you to understand how each solution would be implemented without actually implementing it.
4. Confirm A Solution To Code With Your Interviewer
The next step is to confirm a solution to code with your interviewer. Confirming a solution to code with your interviewer means making sure that you’re both aligned with the solution and approach you’re going to use in solving the coding problem your interviewer provided you with.
This step is important because your interviewer may be able to guide you through certain aspects of a solution that you haven’t thought about yet that would come up while you’re coding. Additionally, it provides good communication signals to your interviewer which is good for your coding interview evaluation.
To approach confirming a solution to code with your interview, try and make a recommendation based on your solution space analysis in terms of time and space complexity. Generally, software engineer coding interviewers will expect you to pick and implement the solution optimized for the best time complexity. However, it’s important to confirm this with your interviewer in case they are evaluating based on a different solution for the purpose of your current interview.
5. Code Your First Solution
The fifth step you must to do solve a coding interview question during an interview is to code your first solution. Coding your first solution means writing working code based on the solution you agreed upon coding with your interviewer.
You’ll want to make sure that you’re communicating with your interviewer throughout the duration that you’re coding your first solution. In fact, this is a part of how you should communicate with your interviewer during a coding interview because you’re trying to maximize the amount of positive feedback that your interviewer can write down about you within their evaluations.
Sometimes, it’s difficult to map a solution to working code, so it might be beneficial to write down brief notes about the solution’s implementation as pseudo code before you start coding. However, you should not spend more than 2 minutes writing pseudo code because you don’t have that much time during the duration of a coding interview (about 20 to 30 minutes of coding time) to code a complete solution.
6. Test Your Code
After you code your first solution, the next step is to test that the code you’ve written for your solved problem is correct. Testing your code means applying inputs into your code and validating that your code outputs are correct. The validation process that your code works as intended is a manual process where you’ll be expected to take a test case and go through your code as if you’re a computer. However, Some companies allow you to run automated test cases in an IDE.
When you’re going through your code to make sure that it works, make sure that you’re tracking the state of your program exactly as it’s written as code. Often, candidates will make the mistake of talking about their code as they intend it to work rather than how it actually works.
To do this, you can track all of the variables and how each variable is changing throughout your program’s execution. Additionally, you can pay close attention to any complex boolean evaluations that are done by your program, making sure to never assume you know the value of an evaluation and computing it every time.
If you find a bug in your code, take some time to consider what needs to be fixed. Identify the broken state of your program and communicate with your interviewer the entire time. You’re not expected to write perfect code when you code your first solution. However, if there are mistakes in your code, it’s a good signal to your interviewer if you’re able to identify all the mistakes and fix them all yourself.
7. Optimize Your Solution
The last step to solving a coding interview problem within a software engineer technical interview is to optimize your solution. Optimizing your solution means looking at your code and making it run as fast as possible using as little memory as possible.
At the optimization stage of solving a technical interview problem, you’re looking for both time and space complexity optimizations and microcode optimizations that are less impactful (like variable position changes and reducing the number of required function calls).
In practice, you’ll likely only need to describe what you would do to optimize your solution to your interviewer at this point because you’ll be near the end of an interview. However, you’ll want to confirm what you should do with your interviewer.
How Do You Optimize A Solution?
To optimize a solution in a coding interview, you’ll want to follow a framework for identifying what can be simplified or moved around for efficiency. A popular framework for optimizing solutions is by following the BUD framework which stands for reducing bottlenecks, unnecessary work, and duplicated work.
Why Is Solving A Coding Interview Problem Not Enough To Crack The Coding Interview?
Solving a coding interview problem is not enough to crack the coding interview because being able to solve a coding interview problem is only one of the criteria in the 3 total criteria for coding interviews. The other criteria that you’re evaluated in a coding interview are communication skills and analytical abilities.
In order to increase your odds of passing a coding interview, you will want to make sure that you make use of all the resources that you can to improve your problem-solving skills, communication skills, and analytical abilities. These resources include books, courses, and mock interview platforms.
What Resources Can Help You Pass Technical Coding Interview?
Resources that you can use to pass a coding interview are listed below.
- Coding Interview Books: Cracking the Coding Interview is an example of a book that explains all of the components of a coding interview that you should know about in order to solve interview problems and pass interviews.
- Coding Interview Courses: AlgoExpert is an example of a coding interview course that provides you with videos that show you how to solve coding interview problems.
- Coding Interview Mock Interview Platforms: Pramp is an example of a free mock coding interview platform that you can use to practice solving coding interview problems.