101 Essential Coding Interview Questions To Prepare For Coding Interviews
Coding interview questions are types of questions that are asked within software engineering interviews to assess a candidate’s ability to communicate about, think through, and solve problems related to data structures and algorithms.
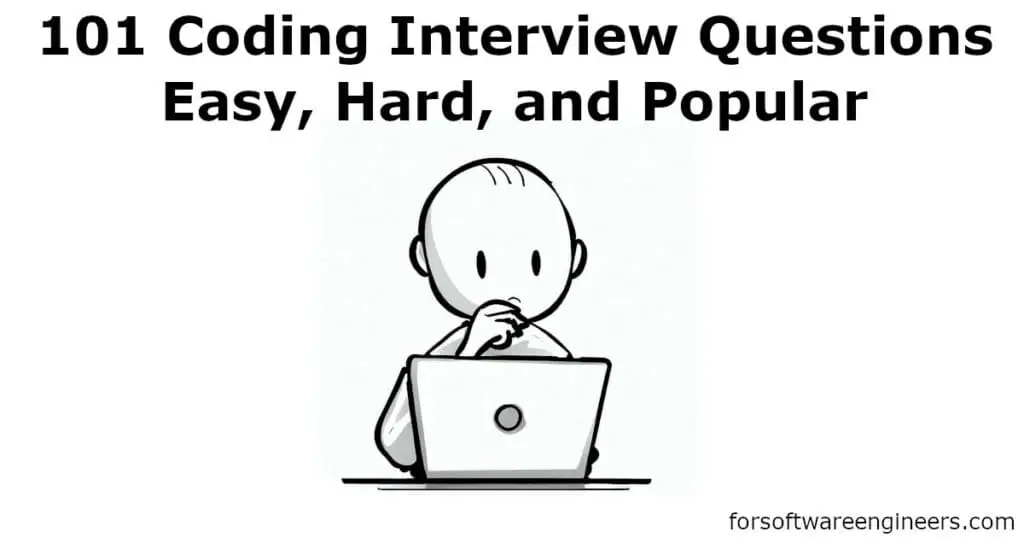
For aspiring software engineers, coding interview questions are one of the most important aspects of the software engineer coding interview that they need to pass in order to get a job as a software engineer. As a result, it’s important to practice as many relevant coding interview questions as you can to prepare for coding interviews that ask coding interview questions.
You can find lists of coding interview questions like “The Blind 75” and “NeetCode 150” if you search on online forums like LeetCode’s solutions pages and TeamBlind. However, in this article, we will be covering 101 essential coding interview questions that we think will better help you pass coding interviews. These lists cover 21 common coding interview questions, 20 easy interview questions, 20 hard coding interview questions, 20 coding questions companies like Google ask, and 20 common conceptual questions that are asked.
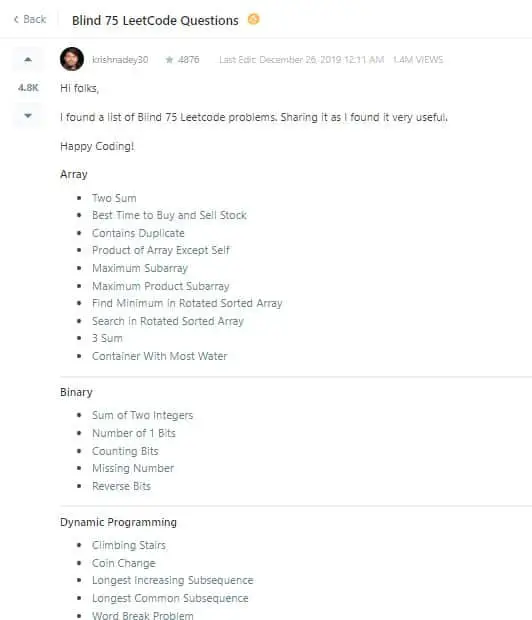
At the end of the article, we’ll discuss the additional things you can do to improve your chances of passing a coding interview like how to approach solving a coding problem, the most important data structures to know, and the most important algorithms to learn. Additionally, we’ll talk about the best resources to learn about coding interview question types and approaches like courses and books.
What Is A Coding Interview Question?
A coding interview question (also known as a coding interview problem) is a type of structured interview question that has one or more solutions that involve creatively using data structures (like stacks, queues, and lists) and algorithms (like binary search and depth-first search) to complete. Coding interview problems require approximately 5 to 30 minutes to complete, depending on the difficulty of the problem which ranges from easy, medium, and hard difficulty levels.
The image below depicts a screenshot of LeetCode, a website that is known for its numerous amount of coding interview questions.
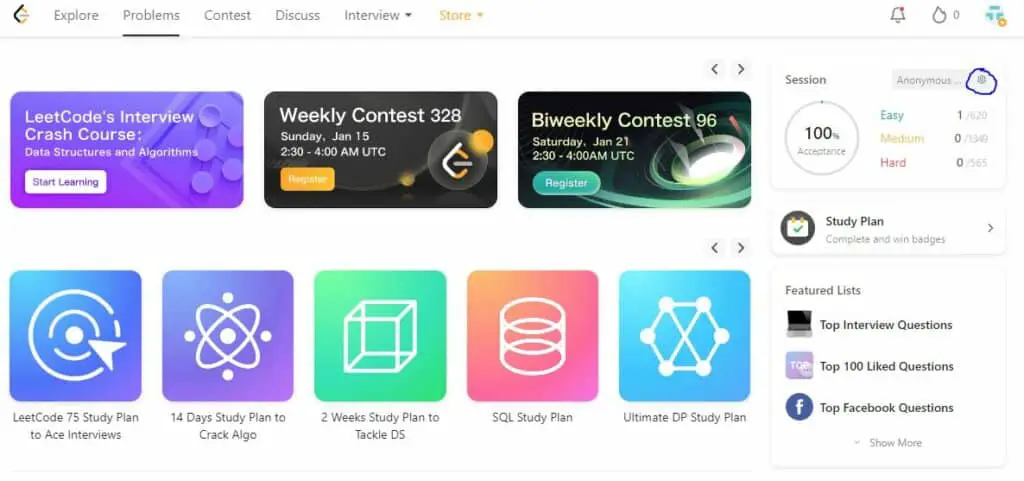
Conceptual coding interview questions are questions related to topics in computer science like data structures, algorithms, and object-oriented programming. These types of questions are asked in a way that requires candidates to describe what concepts are and how they work.
21 Common Coding Interview Questions
Common coding interview questions are questions that frequently appear in coding interviews either verbatim or in variation. These are essential coding interview questions that you should know how to complete if you want to increase your chances of passing a software engineering technical interview.
A list of the 21 most common coding interview questions (from LeetCode) is listed below along with a short description of what the question entails and what the question tests you on.
- FizzBuzz: Write a program that prints numbers from 1 to N, but for multiples of 3, print “Fizz” instead of the number, and for multiples of 5, print “Buzz”. For numbers that are multiples of both three and five, print “FizzBuzz”. This question tests basic programming logic and control flow.
- Reverse a String: Implement a function to reverse a given string. This tests understanding of string manipulation and iteration.
- Find the First Non-Repeated Character: Given a string, find the first character that doesn’t repeat. This assesses knowledge of data structures and string processing.
- Two Sum: Given an array of integers, find two numbers such that they add up to a specific target number. This tests array manipulation and problem-solving skills.
- Palindrome: Determine if a given string is a palindrome (reads the same backward as forward). This gauges understanding of string manipulation and comparison.
- Merge Intervals: Given a collection of intervals, merge any overlapping intervals. This tests sorting and array manipulation skills.
- Linked List Cycle: Determine if a linked list has a cycle in it. This evaluates knowledge of linked lists and Floyd’s cycle-finding algorithm.
- Balanced Parentheses: Check if a string has balanced parentheses. This tests understanding of stack data structure and string processing.
- Binary Search: Implement binary search on a sorted array. This tests knowledge of search algorithms and array manipulation.
- Tree Traversal: Implement in-order, pre-order, and post-order traversal of a binary tree. This evaluates understanding of tree data structures and recursion.
- Maximum Subarray: Find the contiguous subarray with the largest sum. This tests dynamic programming and array manipulation skills.
- Longest Common Prefix: Find the longest common prefix string amongst an array of strings. This tests string processing and comparison skills.
- Anagrams: Group an array of strings so that all anagrams are together. This tests string manipulation and hashing.
- Isomorphic Strings: Determine if two strings are isomorphic (every character in string A can be replaced to get string B). This tests pattern recognition and string processing.
- Depth First Search (DFS) and Breadth First Search (BFS): Implement DFS and BFS for a graph. This evaluates your understanding of graph traversal algorithms.
- Minimum Window Substring: Given two strings S and T, find the minimum window in S which will contain all the characters in T. This tests string processing and the sliding window technique.
- Longest Palindromic Substring: Find the longest palindromic substring in a given string. This tests dynamic programming and string manipulation.
- Valid Sudoku: Determine if a given 9×9 Sudoku board is valid. This tests matrix manipulation and validation skills.
- Implement Trie (Prefix Tree): Implement a trie with insert, search, and startsWith methods. This tests understanding of tree-like data structures and string processing.
- LRU Cache: Design and implement a least recently used (LRU) cache. This evaluates knowledge of data structures and caching mechanisms.
- Shortest Path in a Maze: Given a 2D matrix representing a maze, find the shortest path from a start point to an end point. This tests graph algorithms and matrix manipulation.
20 Easy Coding Interview Questions
Easy coding interview questions are types of coding interview questions that exist for people who are new to solving programming interview problems. These problems are easy and fast to solve and act as introductory problems to intermediate problems.
A list of 20 easy coding interview questions (from LeetCode) is listed below along with a short description of what the question entails and what the question tests you on.
- Array Sum: Calculate the sum of all numbers in an array. This tests basic array traversal and arithmetic operations.
- Count Vowels: Count the number of vowels in a given string. This evaluates basic string processing and character comparison.
- Factorial: Compute the factorial of a given number using recursion. This tests understanding of recursion and mathematical operations.
- Find Maximum: Find the largest number in an array. This gauges basic array traversal and comparison skills.
- Duplicate Elements: Determine if an array contains any duplicate elements. This tests array manipulation and element comparison.
- Nth Fibonacci: Find the Nth number in the Fibonacci sequence. This evaluates understanding of recursion or iterative solutions.
- Array Rotation: Rotate an array to the right by k steps. This tests array manipulation and shifting operations.
- Single Number: Find the number that appears only once in an array where every other number appears twice. This tests bitwise operations and array traversal.
- Valid Anagram: Check if two given strings are anagrams of each other. This evaluates string processing and character count comparison.
- Move Zeroes: Move all zeroes in an array to the end without changing the order of non-zero elements. This tests array manipulation and ordering.
- Hamming Distance: Calculate the Hamming distance between two integers. This evaluates your understanding of bitwise operations.
- Power of Two: Determine if a given number is a power of two. This tests bitwise operations and mathematical properties.
- Palindrome Number: Check if a given integer is a palindrome. This evaluates number manipulation and comparison.
- Valid Mountain Array: Determine if an array is a valid mountain (increases then decreases). This tests array traversal and pattern recognition.
- Majority Element: Find the element that appears more than half the time in an array. This tests array traversal and counting.
- Climbing Stairs: Calculate how many distinct ways one can climb a staircase of N steps, taking 1 or 2 steps at a time. This evaluates understanding of dynamic programming or recursion.
- Island Perimeter: Given a 2D grid of 1s (land) and 0s (water), find the perimeter of the island. This tests matrix traversal and boundary detection.
- Reverse Integer: Reverse the digits of a given integer. This tests number manipulation and overflow detection.
- Contains Duplicate II: Check if an array contains nearby duplicate elements within a given distance k. This evaluates array traversal and element comparison.
- Happy Number: Determine if a number is “happy” (repeatedly summing the square of its digits eventually results in 1). This tests number manipulation and cycle detection.
20 Hard Coding Interview Questions
Hard coding interview questions are types of coding interview questions that exist for people who are familiar with solving programming interview problems. These problems are difficult to solve and are similar to the difficulty of problems that you will see during a real on-site interview at competitive companies.
A list of 20 hard coding interview questions (from LeetCode) is listed below along with a short description of what the question entails and what the question tests you on.
- Serialize and Deserialize Binary Tree: Convert a binary tree to a string and vice versa. This tests understanding of tree traversal and data serialization.
- Longest Increasing Subsequence: Find the length of the longest subsequence of a given sequence that is strictly increasing. This evaluates dynamic programming and sequence analysis.
- Wildcard Matching: Implement wildcard pattern matching with support for ‘?’ and ‘*’. This tests string manipulation and dynamic programming.
- Maximal Rectangle: Given a 2D binary matrix, find the largest rectangle containing only 1s. This evaluates matrix traversal and stack usage.
- Sliding Window Maximum: Find the maximum value in each subarray of size k in an array. This tests deque data structure and window traversal.
- Minimum Window Subsequence: Given strings S and T, find the minimum window in S which will contain all characters of T in order. This evaluates string processing and the two-pointer technique.
- Distinct Subsequences: Count the number of distinct subsequences of string S that equal string T. This tests dynamic programming and string manipulation.
- Trapping Rain Water: Calculate how much rainwater can be trapped between bars of different heights. This evaluates the array traversal and two-pointer technique.
- Burst Balloons: Given an array of numbers, find the maximum points you can obtain by bursting balloons wisely. This tests dynamic programming and interval partitioning.
- LRU Cache II: Design a data structure that supports the LRU cache with O(1) operations. This evaluates the understanding of data structures and caching mechanisms.
- Alien Dictionary: Given a sorted dictionary of an alien language, find the order of characters. This tests graph construction and topological sorting.
- Shortest Palindrome: Find the shortest palindrome by adding characters in front of a given string. This tests string manipulation and the KMP algorithm.
- Max Points on a Line: Given 2D points, find the maximum number of points that lie on the same straight line. This evaluates geometry and hash table usage.
- Regular Expression Matching: Implement regular expression matching with support for ‘.’ and ‘*’. This tests string processing and dynamic programming.
- Number of Islands II: Given a 2D grid and a list of positions, find the number of islands after each position is added. This evaluates union-find data structure and matrix manipulation.
- Minimum Sliding Window: Find the smallest window in a string that contains all characters of another string. This tests string manipulation and the sliding window technique.
- Longest Consecutive Sequence: Find the length of the longest consecutive sequence in an unsorted array. This evaluates array manipulation and hash set usage.
- Palindrome Pairs: Given a list of words, find all pairs of unique indices such that the concatenation of the two words is a palindrome. This tests string manipulation and hash table usage.
- Skyline Problem: Given the positions and heights of buildings, compute the skyline. This evaluates divide and conquer and event sorting.
- Word Ladder II: Find all shortest transformation sequences from a start word to an end word, given a word list. This tests breadth-first search and path reconstruction.
20 Coding Interview Questions For Companies Like Google and Amazon
Coding interview questions for companies like Google and Amazon are a mix of medium to hard coding interview questions. They’re similar in rigor to what you would view in a phone or onsite interview at FAANG companies.
A list of 20 coding interview questions (from LeetCode) for preparing for FAANG companies is listed below along with a short description of what the question entails and what the question tests you on.
- Kth Largest Element: Find the kth largest element in an unsorted array. This tests understanding of sorting algorithms and selection algorithms.
- Top K Frequent Elements: Find the k most frequent elements in an array. This evaluates proficiency with hash maps and priority queues.
- Minimum Spanning Tree: Construct the minimum spanning tree for a given graph. This tests knowledge of graph algorithms like Kruskal’s or Prim’s.
- Copy Random List: Clone a linked list where each node has a random pointer. This evaluates deep copy techniques and linked list manipulation.
- Word Break: Determine if a string can be segmented into space-separated words from a dictionary. This tests dynamic programming and string manipulation.
- Search in Rotated Sorted Array: Search for a target in a rotated sorted array. This evaluates binary search adaptations and array manipulation.
- Meeting Rooms II: Find the minimum number of conference rooms required given a list of meeting intervals. This tests interval sorting and heap usage.
- Design a URL Shortener: Design a system that takes long URLs and converts them into short and unique ones. This evaluates system design and hashing techniques.
- Find Median from Data Stream: Implement a data structure to find the median of a stream of numbers. This tests understanding of heaps and dynamic data stream processing.
- Course Schedule: Determine if it’s possible to finish all courses given prerequisites. This evaluates topological sorting and graph traversal.
- Largest Rectangle in Histogram: Find the largest rectangle that can be formed in a histogram. This tests stack usage and array traversal.
- Substring with Concatenation of All Words: Find all starting indices of substring(s) that is a concatenation of each word in a list exactly once. This evaluates the sliding window technique and string manipulation.
- Design Tic-Tac-Toe: Design a Tic-Tac-Toe game that can be played between two players on an n x n grid. This tests game logic and system design.
- Minimum Window Subsequence II: Given strings S and T, find the minimum contiguous subsequence in S that contains all characters of T in order. This tests the two-pointer technique and string processing.
- Design Search Autocomplete System: Design a search autocomplete system for a search engine. This evaluates trie data structures and system design.
- Zombie in Matrix: Given a 2D grid, each cell is either a zombie or a human, determine the minimum days required to convert all humans into zombies. This tests breadth-first search and matrix traversal.
- Design a Rate Limiter: Implement a rate limiter for an API. This evaluates system design and sliding window or token bucket techniques.
- Maximal Network Rank: Given a network of cities and roads, find the maximum network rank between two cities. This tests graph analysis and combinatorics.
- Design Twitter: Design a simplified version of Twitter where users can post tweets and follow/unfollow other users. This evaluates system design, data structures, and object-oriented principles.
- Implement Trie II: Implement a trie supporting insert, search, and delete operations. This tests understanding of tree-like data structures and string processing.
20 Common Conceptual Coding Interview Questions
Conceptual coding interview questions are questions that don’t require programming to solve. Typically, conceptual questions during a coding interview as initial and/or follow-up questions to the problem being solved. These questions require a verbal answer that is evaluated by an interviewer, instead of an answer that’s written down in code.
A list of 20 conceptual coding interview questions (from LeetCode) is listed below along with a short description of what the question entails and the importance of the question.
- Big O Notation: Explain the concept of Big O notation. It measures the worst-case time complexity of algorithms, crucial for evaluating performance.
- Recursion vs. Iteration: Differentiate between recursion and iteration. Understanding both approaches helps in solving problems with optimal solutions.
- Mutable vs. Immutable: Define mutable and immutable objects. This is essential for understanding data modification and its implications in programming.
- Pass by Value vs. Pass by Reference: Explain the difference between passing by value and passing by reference. It’s vital for understanding how data is manipulated in functions.
- Concurrency vs. Parallelism: Differentiate between concurrency and parallelism. This is key for optimizing tasks that can run simultaneously.
- Stack vs. Heap: Describe the difference between stack and heap memory. Understanding memory allocation helps in efficient programming and avoiding leaks.
- Trees vs. Graphs: Differentiate between trees and graphs. Both are foundational data structures used in various algorithms and applications.
- Hashing: Explain the concept and purpose of hashing. Hashing is fundamental for data retrieval operations and understanding data structures like hash maps.
- Garbage Collection: Describe how garbage collection works. It’s essential for memory management and preventing memory leaks in certain languages.
- Depth-First vs. Breadth-First Search: Differentiate between DFS and BFS algorithms. Both are fundamental graph traversal techniques with different use cases.
- Dynamic Programming: Explain the principle behind dynamic programming. It’s a method for solving complex problems by breaking them down into simpler subproblems.
- Object-Oriented Principles: Describe the main principles of object-oriented programming. These principles are foundational for designing robust and scalable software systems.
- Database Normalization: Explain the concept of database normalization. It’s crucial for designing efficient databases that minimize redundancy.
- CAP Theorem: Describe the CAP theorem and its implications. It’s vital for understanding trade-offs in distributed systems.
- RESTful Services: Explain the principles behind RESTful web services. REST is a standard for designing networked applications.
- MVC Architecture: Describe the Model-View-Controller architecture. MVC is a design pattern used in web development for separating concerns.
- Microservices: Explain the microservices architecture and its benefits. Microservices are essential for building scalable and maintainable systems.
- Functional Programming: Describe the principles of functional programming. It’s a programming paradigm that treats computation as the evaluation of mathematical functions.
- Polymorphism: Explain the concept of polymorphism in programming. It’s a fundamental object-oriented principle that allows objects of different types to be treated as if they are objects of the same type.
- Caching Mechanisms: Describe the purpose and types of caching in software systems. Caching improves performance by storing frequently used data in a manner that allows faster access.
How Do You Improve Your Chances Of Passing A Coding Interview?
To improve your chances of passing a coding interview beyond just practicing coding questions, you’ll need to follow a successful process for solving coding interview problems, understand the most important data structures, and learn about the most important algorithms for coding interviews. You should also prepare for coding interviews through resources such as mock interviews, courses, and books.
What Is The Best Way To Solve An Coding Interview Problem?
The best way to solve a coding interview problem is to practice following a methodological process to solve coding interview questions. With enough practice solving coding interview problems with a good process, you’ll be able to give your interviewers the most amount of positive evaluation signals, increasing your chances of passing a coding interview.
What Are The Most Important Data Structures For Technical Interviews?
There are 9 most important data structures to study for coding interviews which include strings, arrays, hash tables, stacks, queues, linked lists, trees, heaps, and graphs.
Studying each of these data structures, understanding their use cases, and knowing how and when to apply them will improve your chances of passing the vast majority of coding interviews. These data structures are the 9 most important data structures to study for a coding interview because they’re the most common data structures that are used in optimal solutions for coding interview problems.
What Are The Most Important Algorithms For Technical Interviews?
The most important algorithms to study for a coding interview are insertion sort, depth-first search (DFS), breadth-first search (BFS), and binary search.
Many coding interview problems have optimal solutions related to these most important algorithms. However, to significantly improve your chances of passing coding interviews, you’ll want to study all 6 types of essential algorithms.
The 6 types of algorithms you should study for a technical interview include sort algorithms, searching algorithms, recursive algorithms, divide-and-conquer algorithms, tree traversal algorithms, graph algorithms, and sliding window algorithms.
What Are The Best Ways Of Practicing Coding Interviews Questions?
The best ways of practicing coding interviews are by practicing yourself on platforms like LeetCode, through doing mock interviews on platforms like Pramp, taking a course on passing coding interviews on platforms like AlgoExpert, and reading books like Cracking the Coding Interview.
What Are The Best Places To Practice Mock Interview Questions?
The best places to practice mock interview questions are websites like Pramp and Interviewing.io. Pramp offers a free solution to mock interviews by pairing you up with peers to conduct mock interviews, and Interviewing.io offers a paid solution for you to hire professional mock Interviewers from companies like Apple, Amazon, Google, and Netflix.
What Are The Best Courses For Getting Better At Coding Interview Questions?
The best courses to get better at solving coding interview questions are Interview Cake and AlgoExpert. These courses are some of the highest-rated courses on learning to solve coding interview questions.
What Are The Best Books For Getting Better At Coding Interview Questions?
The best books for getting better at solving coding interview questions are Cracking the Coding Interview and Elements of the Programming Interview. These books are known as the best books for learning about how to approach coding interviews and coding interview questions.