9 Essential Data Structures For Coding Interviews
A data structure is a specialized framework that dictates how data is stored, manipulated, and retrieved. Various data structures are designed to represent distinct types of data within a program. Each structure offers unique use cases, advantages, and disadvantages toward time and space complexity.
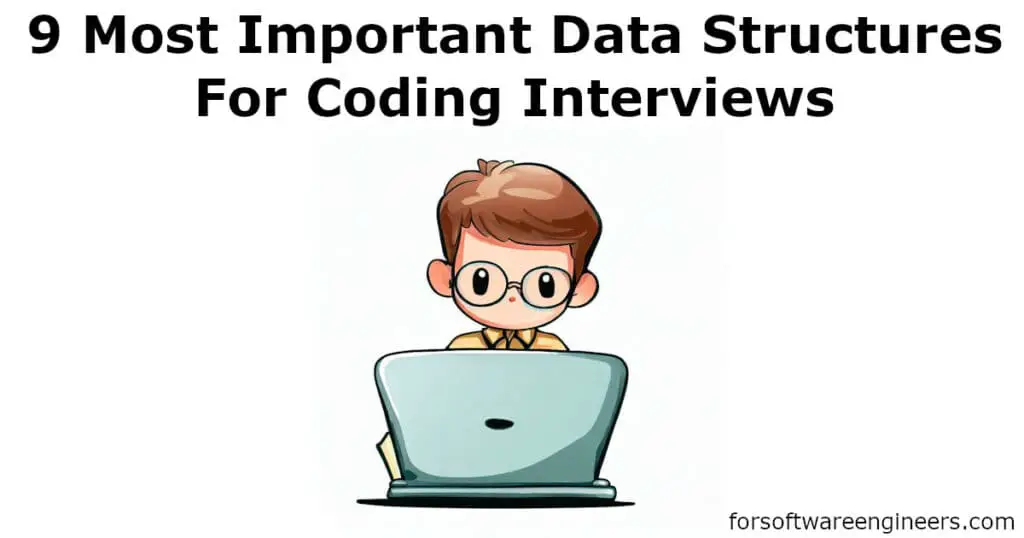
A coding interview tests your knowledge of commonly used and known data structures because it’s important knowledge to have as a software engineer. So, that’s why it’s important to study the 9 most commonly used data structures in coding interviews which are strings, arrays, hash tables, stacks, queues, linked lists, trees, heaps, and graphs.
In this article, we will discuss each of these essential data structures for coding interviews in detail, explain their significance, and show a diagram of what the data structure looks like visually. At the end of the article, we will discuss additional tips you can use to prepare for data structure-related technical interviews for software engineer roles.
1. String
A string is a data structure that is used to represent an ordered sequence of characters. Strings are typically implemented as immutable data structures that have underlying implementations that use arrays to store their characters. This means that strings are inefficient (linear time complexity) data structures to use if you want to effectively mutate the values a String contains because the mutation would require a clone of the string to be created.
Strings are a prerequisite data structure to learn and understand how to use for the vast majority of coding interview problems. They’re as essential to know about as other primitives in programming such as booleans, integers, and floats.
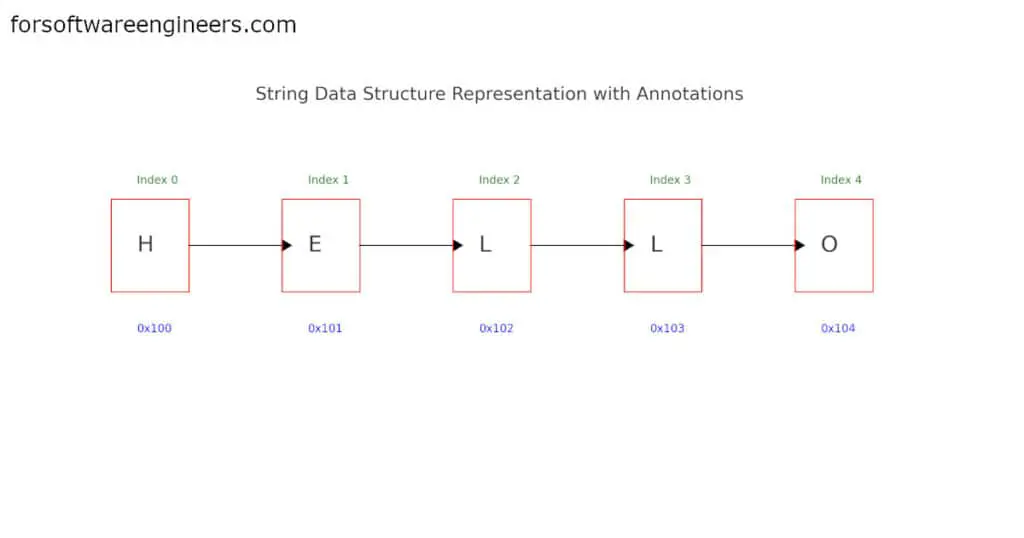
2. Array
An array is a fixed-size data structure that is used to represent an ordered sequence of same-type objects or primitives. Arrays are highly efficient (constant time complexity) for accessing and manipulating values contained within them because of how they’re stored in contiguous memory locations.
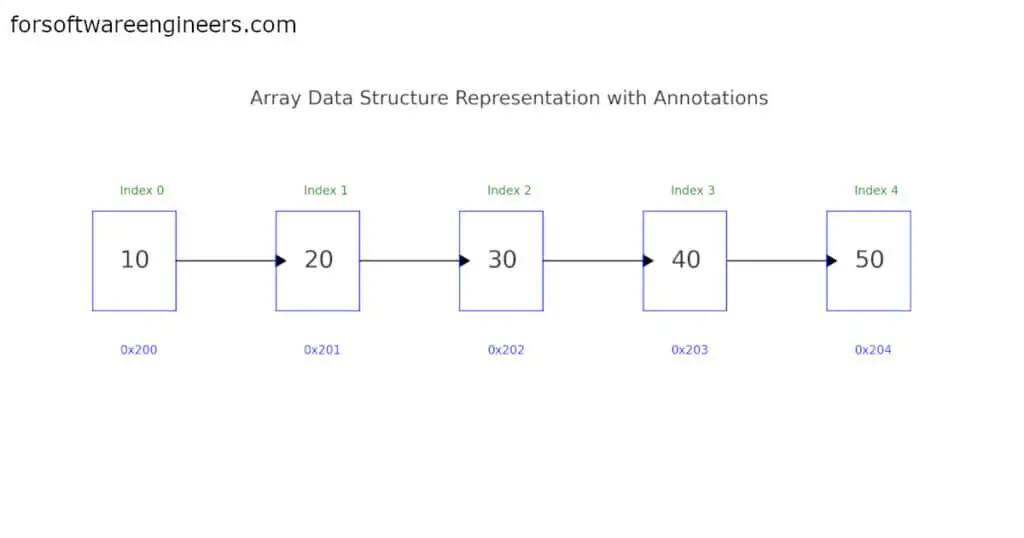
A multidimensional array (also known as a matrix) is an array that contains arrays within it as elements. For instance, a 2D array has 2 levels of arrays within it, and a 3D array has 3 levels of arrays within it.
Arrays are important data structures for coding interview problems because they’re commonly used as 2D matrixes to represent scenarios in complex coding interview problems such as mazes.
3. Hash Table
A hash table is a data structure that stores key-value pairs and allows you to get, put, and replace key-value pairs based on keys in a highly efficient (constant time complexity) way. It achieves this by using a hash function on the keys to determine where they should be stored in memory, usually implemented as an array.
Hash Tables are an important data structure for coding interviews because they’re commonly used as a part of an optimal solution to a technical problem. A common joke within coding interview preparation is that you should throw a hash table at a problem if you’re stuck and don’t know how to solve it because it’ll often lead you to the optimal solution.
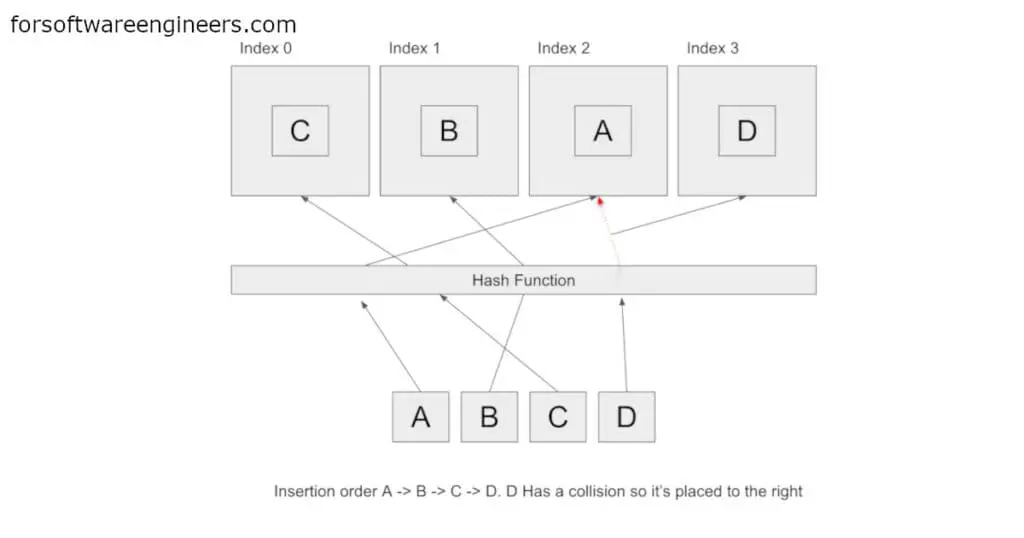
4. Stack
A stack is a data structure that allows you to store and retrieve data from it in a Last-In-First-Out (LIFO) methodology. This means that you can add an element onto the top of a stack (known as “pushing”), view the top element of the stack (known as “peeking”), and remove the element on top of the stack (known as “popping”). Adding an element into a stack, removing an element from the top of a stack, and looking at an element on top of a stack are efficient data structure operations (constant time complexity).
Stacks are essential data structures to learn and study for coding interviews because they’re commonly used when interviewers require you to code a recursive solution in an iterative manner. The stack data structure is used to maintain the execution state of your program and simulate the recursive call stack.
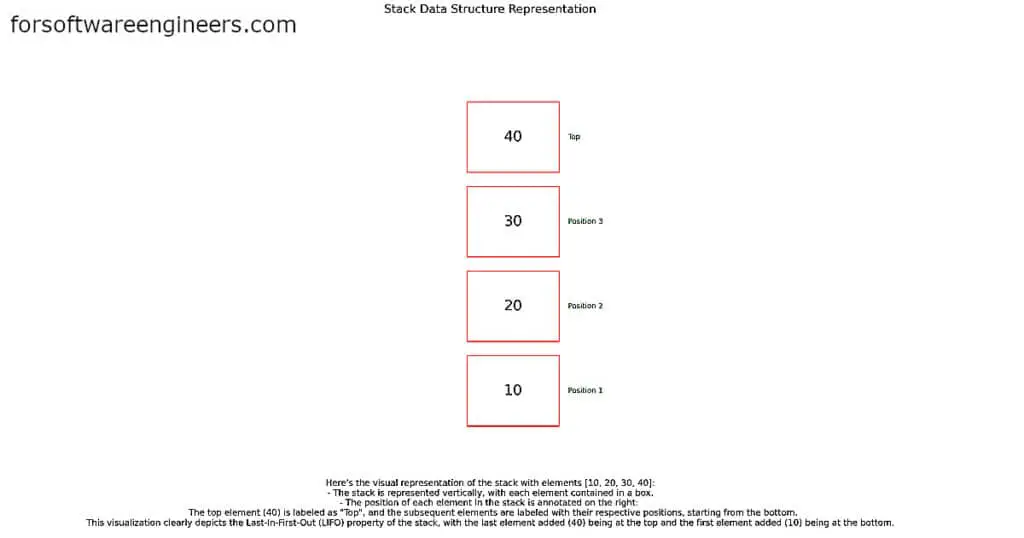
5. Queue
A queue is a data structure that allows you to store and retrieve data from it in a First-In-First-Out (FIFO) methodology. This means that you can add an element to the back of a queue (known as “offering”), view the front of the queue (known as “peeking”), and remove the element in the front of the queue (known as “polling”). Adding an element into a queue, removing an element from a queue, and looking at the front of a queue are efficient data structure operations (constant time complexity).
The queue is an important data structure to study for coding interviews because they’re useful for tasks such as performing breadth-first searches. They’re also useful for representing min heaps and max heaps if you use specialized types of queue data structures like priority queues.
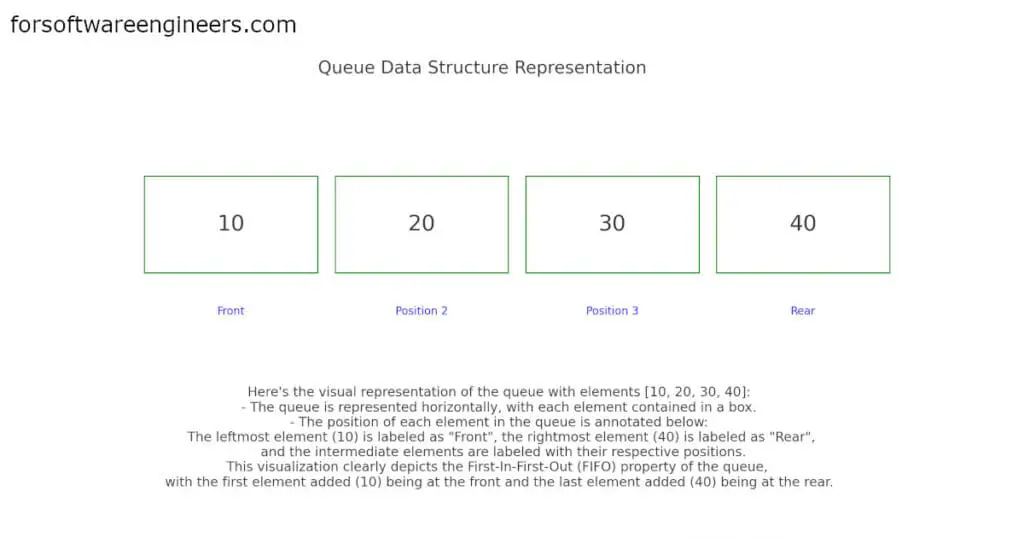
6. Linked List
A linked list is a data structure that is made up of an orchestration of nodes that contain data and point either unidirectionally (singly linked lists) or bidirectional (doubly linked lists) at each other. Linked lists offer efficient operations for adding elements to the end of the list (constant time complexity), however, adding and removing elements is less efficient (linear time complexity).
A linked list data structure is important to know for coding interviews because they’re commonly used data structures in screening interviews like phone interviews. There are a handful of common patterns that are used to solve the vast majority of linked list problems, so you’ll be able to solve this entire class of problems if you learn and understand how to use and manipulate pointers within linked lists.
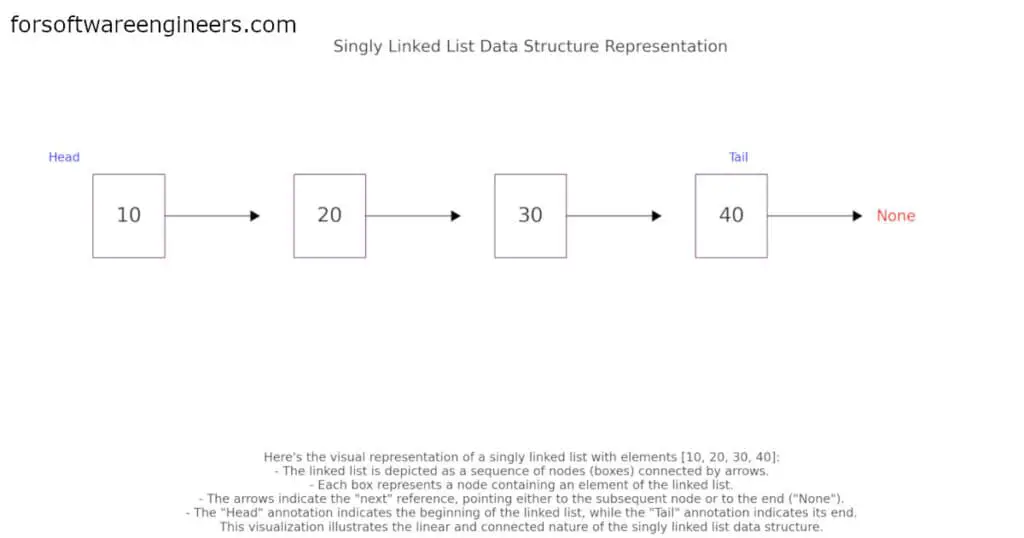
7. Tree
A tree is a data structure a type of data structure that arranges nodes in a way that allows them to have the property of having child nodes accessible through edges. In the case of a binary tree, a node can have up to 2 total child nodes (a left node and a right node). The root of the tree is the top of the tree. Searching for an element and adding an element to a tree is efficient (logarithmic time complexity) if the tree is balanced, otherwise it’s inefficient (linear time complexity).
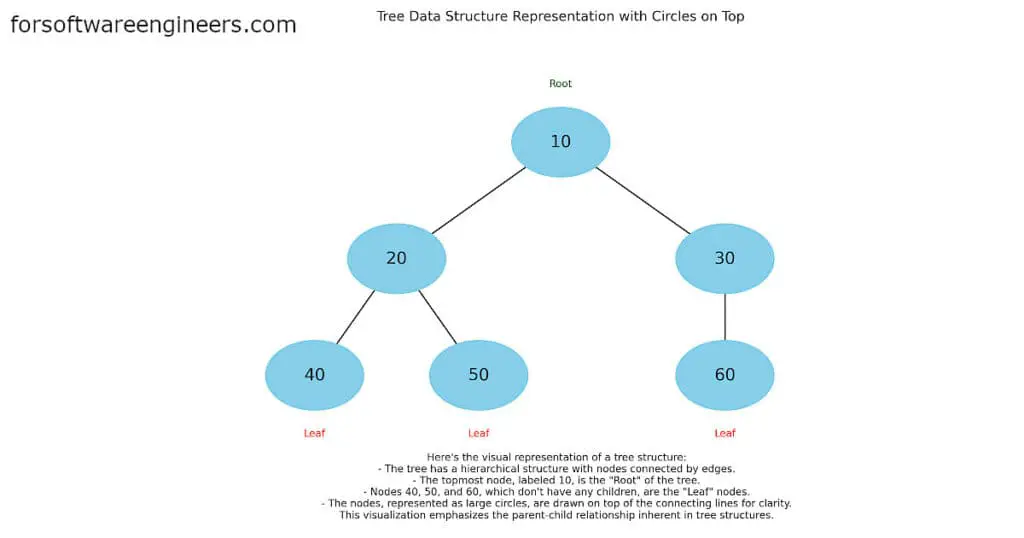
A tree is a common data structure that is used throughout all coding interview rounds. Traversal through trees in a way that solves a problem optimally is challenging and tricky for interviewees who haven’t practiced enough tree problems. This is why it is important to make sure that you practice enough variations of tree-related data structure problems before your coding interviews.
8. Heap
A heap is a data structure that is a type of binary tree with the unique property of maintaining “the heap property”. The heap property ensures that each node is greater than or equal to its child nodes in max-heap, and the heap property ensures that each node is less than or equal to its child nodes in a min-heap. Similar to a queue you can offer elements into a heap (logarithmic time complexity) and remove elements from a heap (also logarithmic time complexity).
Heaps aren’t common data structures that are used in solutions to coding interview questions, however, they’re a part of the most optimal solutions when they are necessary. As a result, it’s important to practice using the heap data structure in heap-related data structure problems.
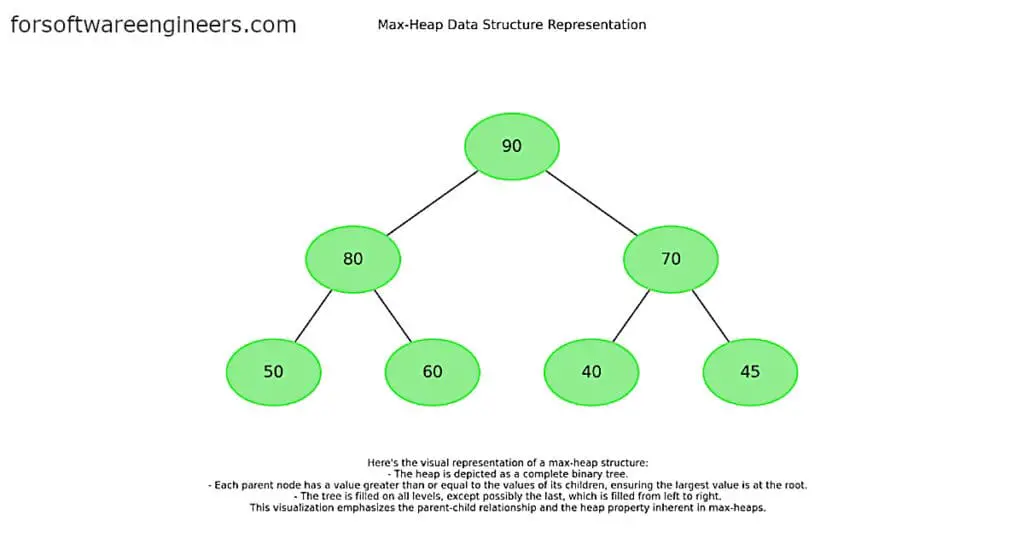
9. Graph
A graph is a data structure that is comprised of vertices (also called nodes) that are connected to each other through edges. The edges can be either unidirectional or bidirectional, and the edges can be weighted or unweighted.
Graph-related data structure problems are commonly asked during difficult coding interviews for companies with bars for hiring like Google, Meta, Amazon, Amazon, and Microsoft. Usually, graph-related data structure coding interview problems have solutions that require you to use other data structures to solve like stacks and queues in addition to advanced algorithms like the Dijkstra shortest path algorithm.
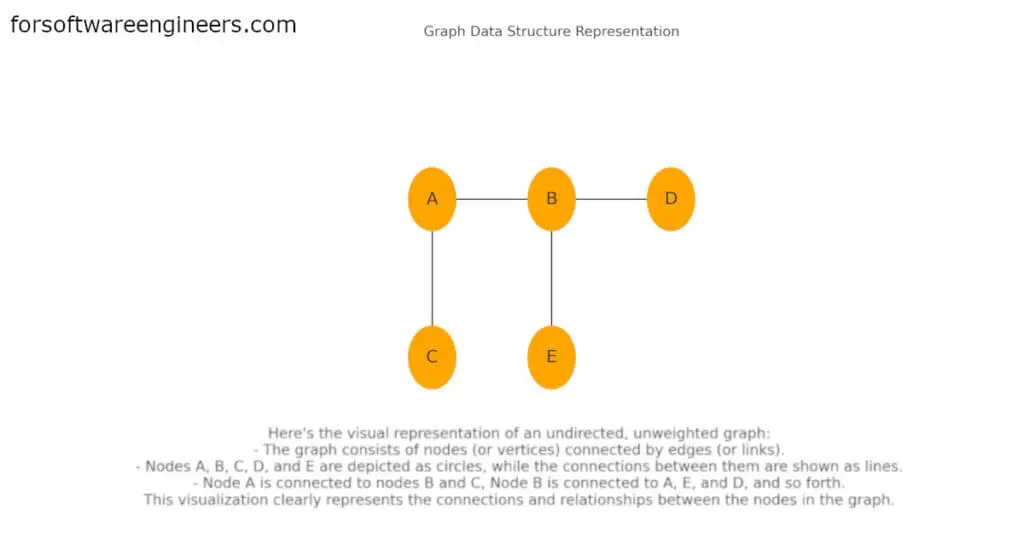
How Do You Prepare For Data Structures-Focused Coding Interviews?
You prepare for data structure-focused coding interviews by practicing coding interview questions that are related to both data structures and algorithms. Knowledge of common data structures and algorithms is an essential aspect of solving coding interview problems and passing coding interviews.
What Are The Best Sites To Practice Data Structure Coding Interview Practice Problems
The best websites to practice data structure coding interview problems include websites like LeetCode and HackerRank. Both of these websites allow you to practice the most relevant coding interview questions for coding interviews for free on their online coding platforms which also provide tests for your solutions.
How Important Is Studying Algorithms With Data Structures?
Studying algorithms with data structures is highly important to prepare for coding interviews. You should study the most important algorithms along with data structures if you want to pass your coding interviews. The most important algorithms to study for a coding interview are insertion sort, depth-first search (DFS), breadth-first search (BFS), and binary search.
Many coding interview problems have optimal solutions related to these most important algorithms. However, to significantly improve your chances of passing coding interviews, you’ll want to study all 6 types of essential algorithms.
The 6 types of algorithms you should study for a technical interview include sort algorithms, searching algorithms, recursive algorithms, divide-and-conquer algorithms, tree traversal algorithms, graph algorithms, and sliding window algorithms.